できること
・リッチメニューからLIFFページで会員入力フォームを起動し、入力した会員情報をDX-LINEの友だち(bfml__FmlLineMember__c)に追記する。
・会員フォームの項目と友だちの項目をセットで任意追加することは可能です。
設定方法
① LIFF用のページを公開したVFページで作成する。
② リッチメニューにLIFF URLを設定する。
① 以下のソースコード参考に会員フォームのVFページとApexコントロールを作成する。
VF例:
<apex:page controller="FmlUpdateMemberInfo" showHeader="false" sidebar="false" standardStylesheets="false">
<html>
<head>
<meta charset="UTF-8" />
<!-- ビューポート設定:全画面表示、スケール固定 -->
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no, viewport-fit=cover" />
<title>会員登録フォーム(サンプル)</title>
<style>
/* html, body を全画面表示 */
html, body {
margin: 0;
padding: 0;
width: 100%;
height: 100%;
background-color: #f7f7f7;
}
/* 中央配置のフォームコンテナ */
.container {
max-width: 600px;
width: 100%;
margin: 0 auto;
background-color: #fff;
padding: 20px;
box-sizing: border-box;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
border-radius: 5px;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
h1 {
text-align: center;
margin-bottom: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
input, textarea {
width: 100%;
padding: 8px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
/* エラー時のスタイル */
input.error, textarea.error {
border: 2px solid red;
}
button {
width: 100%;
padding: 10px;
background-color: #28a745;
color: #fff;
border: none;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
}
button:hover {
background-color: #218838;
}
</style>
</head>
<body>
<div class="container">
<h1>会員登録フォーム</h1>
<div id="messages"></div>
<form id="registrationForm">
<div>
<label for="nameInput">名前<span style="color:red;">*</span>:</label>
<input type="text" id="nameInput" name="nameInput" placeholder="名前を入力してください" required="true"/>
</div>
<div>
<label for="ageInput">年齢:</label>
<input type="number" id="ageInput" name="ageInput" placeholder="年齢を入力してください"/>
</div>
<div>
<label for="addressInput">住所:</label>
<input type="text" id="addressInput" name="addressInput" placeholder="住所を入力してください"/>
</div>
<div>
<label for="emailInput">メールアドレス<span style="color:red;">*</span>:</label>
<input type="email" id="emailInput" name="emailInput" placeholder="メールアドレスを入力してください" required="true"/>
</div>
<div>
<label for="phoneInput">電話番号:</label>
<input type="tel" id="phoneInput" name="phoneInput" placeholder="電話番号を入力してください"/>
</div>
<!-- LIFFで取得したuserIdを保持するhidden項目 -->
<input type="hidden" id="hiddenUserId" name="hiddenUserId" />
<div>
<button type="button" onclick="updateMemberInfoJs();">登録</button>
</div>
</form>
</div>
<!-- LIFF SDK の読み込み -->
<script src="https://static.line-scdn.net/liff/edge/2/sdk.js"></script>
<script>
// LIFFの初期化 LIFF IDを置換する
liff.init({ liffId: "2006799359-vkM5xxxQ" })
.then(function() {
// 初期化後にユーザプロフィールを取得
liff.getProfile()
.then(function(profile) {
var userId = profile.userId;
// hidden項目にuserIdをセット
document.getElementById('hiddenUserId').value = userId;
})
.catch(function(err) {
console.error('ユーザプロフィールの取得エラー: ' + err);
});
})
.catch(function(err) {
console.error('LIFFの初期化エラー: ' + err);
});
// 登録処理
function updateMemberInfoJs() {
// エラー表示のリセット
document.getElementById('nameInput').classList.remove("error");
document.getElementById('emailInput').classList.remove("error");
// 必須項目(名前とメールアドレス)の取得とチェック
var nameValue = document.getElementById('nameInput').value.trim();
var emailValue = document.getElementById('emailInput').value.trim();
var hasError = false;
if (!nameValue) {
document.getElementById('nameInput').classList.add("error");
hasError = true;
}
if (!emailValue) {
document.getElementById('emailInput').classList.add("error");
hasError = true;
}
if (hasError) {
// エラーがある場合は処理を中断
return;
}
// その他の入力項目の値を取得
var ageValue = document.getElementById('ageInput').value.trim();
var addressValue = document.getElementById('addressInput').value.trim();
var phoneValue = document.getElementById('phoneInput').value.trim();
var lineUserId = document.getElementById('hiddenUserId').value;
// メンバー情報オブジェクトの作成
// ★オブジェクト「友だち(bfml__FmlLineMember__c)」の項目APIと一致すること、必要に応じて追加する。
let memberObj = {
bfml__Name__c: nameValue,
bfml__Age__c: ageValue,
bfml__Address__c: addressValue,
bfml__Mail__c: emailValue,
bfml__MobileTel__c: phoneValue,
bfml__IsMemberInfoRegistered__c : true, //会員登録済み メール認識URL送信トリガになる
Id: lineUserId
};
let fieldValues = {
dealCommand: 'line_member_update',
channelid: '1657924039', //channelid ★チャンネルID変更する
dealUserKey: lineUserId,
dealContent: JSON.stringify(memberObj)
};
var memberJson = JSON.stringify(fieldValues);
// ApexのRemote Action メソッドを呼び出し
// 以下新規作成したApexクラスを呼び出す
FmlUpdateMemberInfo.updateMemberInfo(memberJson, function(result, event) {
if (event.status) {
// 登録成功:友だちへメッセージ送信後、LIFFページを閉じる
liff.sendMessages([{
type: 'text',
text: '情報登録ありがとうございます' //フォーム情報入力後の送信するメッセージ
}])
.then(function() {
liff.closeWindow();
})
.catch(function(error) {
console.error('メッセージ送信エラー:', error);
liff.closeWindow();
});
} else {
alert('エラー: ' + event.message);
}
}, { escape: true });
}
</script>
</body>
</html>
</apex:page>
Apex:
global without sharing class FmlUpdateMemberInfo {
@RemoteAction
global static String updateMemberInfo(String fieldValuesJson){
return bfml.FmlLineWebHookCallback.insertForLineMember(fieldValuesJson);
}
}
② 上記のVFページをサイトに公開し、LIFFのエンドポイントURLに登録する。

③ LIFF URLをリッチメニューのボタンに登録する。
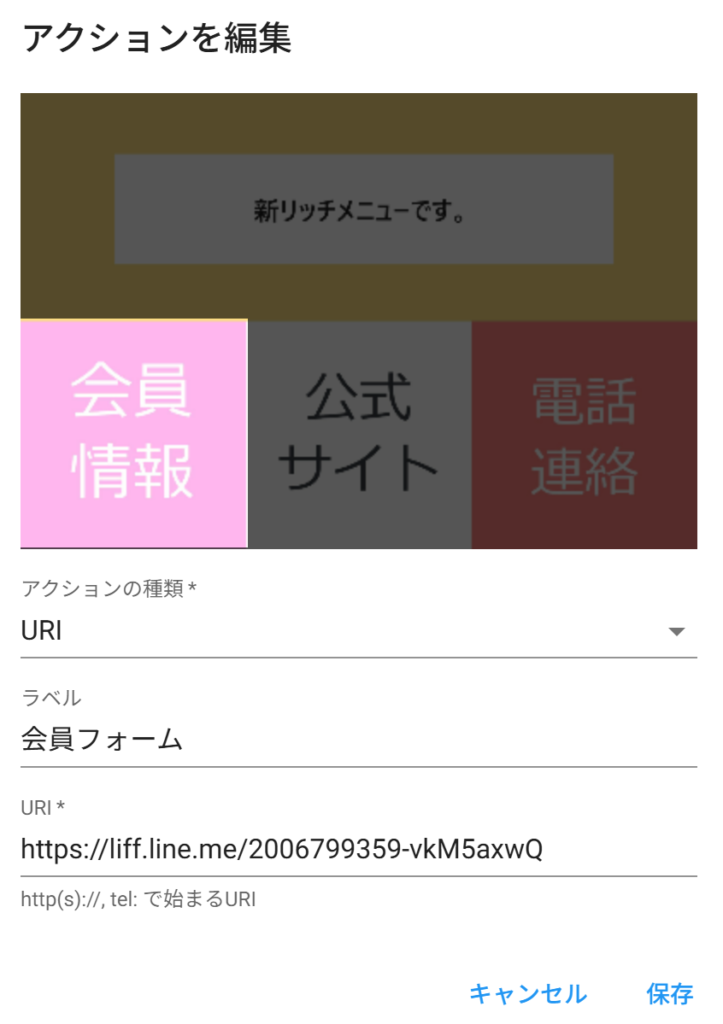